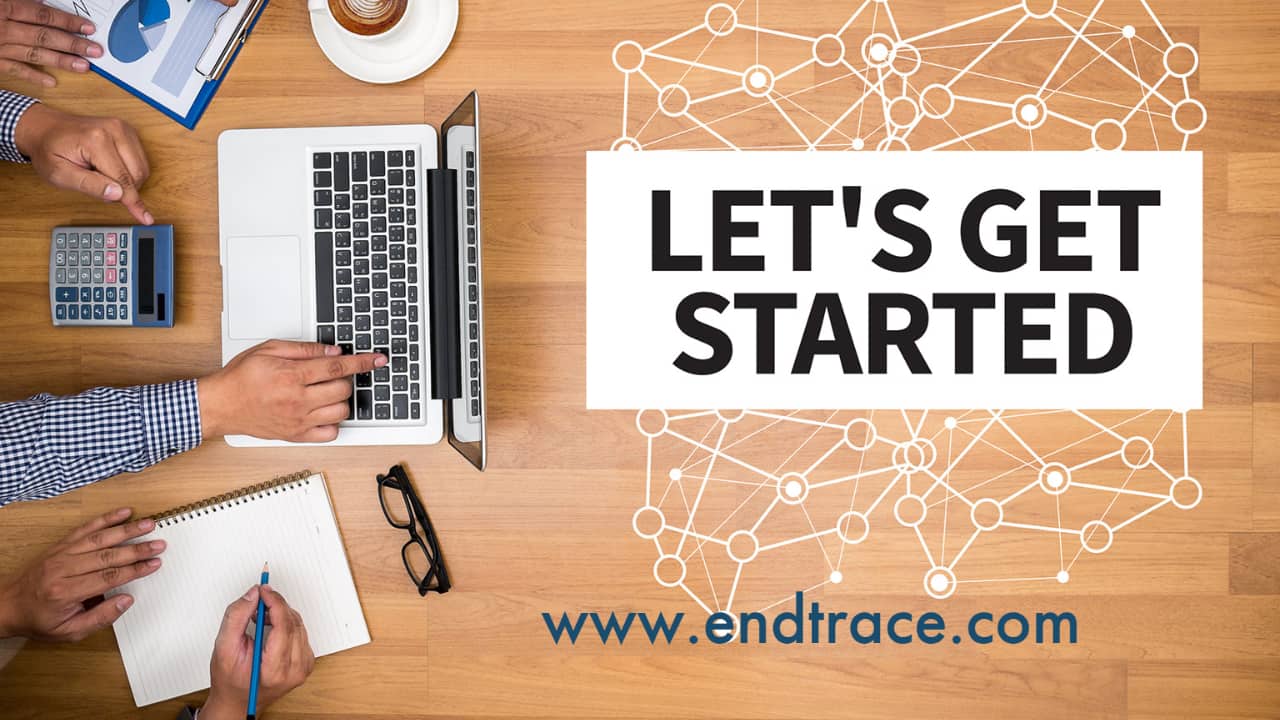
Introduction to Selenium
A. Definition and Brief History
Selenium is a popular open-source automation testing tool that is widely used by developers and testers for automating web-based applications. It was first developed by Jason Huggins in 2004 as an internal tool at ThoughtWorks, and later it was released as an open-source tool in 2008. Since then, Selenium has become one of the most popular automation testing tools in the industry, and it has been adopted by companies of all sizes and industries.
B. Why Use Selenium?
Selenium offers a wide range of benefits to developers and testers, making it a preferred choice for automation testing. Some of the key benefits of using Selenium are:
- It is an open-source tool and is available for free.
- It supports multiple programming languages such as Java, Python, C#, etc.
- It supports multiple browsers such as Chrome, Firefox, Safari, etc.
- It provides a user-friendly interface for creating and executing test scripts.
- It supports parallel test execution, making it suitable for large-scale automation testing projects.
- It supports integration with other tools and frameworks such as TestNG, JUnit, etc.
C. Different Components of Selenium
Selenium is comprised of several components that work together to automate the testing process. The main components of Selenium are:
Selenium IDE: It is a record and playback tool that allows testers to create and execute test cases without any programming knowledge.
Selenium WebDriver: It is a powerful tool for automating web applications, and it provides a programming interface for creating and executing test scripts.
Selenium Grid: It is a tool for running tests in parallel across multiple browsers and operating systems.
D. Prerequisites for Learning Selenium
Before learning Selenium, it is important to have a good understanding of programming concepts and languages such as Java, Python, C#, etc. It is also recommended to have a good understanding of HTML, CSS, and JavaScript as these are the building blocks of web applications. Additionally, it is important to have a basic understanding of software testing concepts and methodologies. With these prerequisites in place, anyone can learn Selenium and become proficient in automation testing.
Getting Started with Selenium
A. Installation and Setup of Selenium with Java
To get started with Selenium, the first step is to install and set up the necessary software components. For Java-based Selenium automation, the following components need to be installed:
- Java Development Kit (JDK)
- Integrated Development Environment (IDE) such as Eclipse, IntelliJ IDEA, etc.
- Selenium WebDriver Java bindings
Once these components are installed, the next step is to configure the environment variables and set up the project in the IDE.
B. Installing Selenium IDE
Selenium IDE is a record and playback tool that allows testers to create test cases without any programming knowledge. It can be easily installed as a browser extension for Google Chrome or Firefox. Once installed, testers can use it to record and play back test cases, export the test cases in different programming languages, and save them for later use.
C. Creating a Basic Selenium Test Script
To create a basic Selenium test script, testers need to follow the below steps:
- Launch the IDE and create a new Java project.
- Add the Selenium WebDriver Java bindings to the project.
- Create a new Java class and define the test script.
- Use the WebDriver interface to navigate to a web page, interact with the elements on the page, and perform actions such as clicking buttons, filling out forms, etc.
- Save the test script and run it to verify the functionality.
- D. Running the Test Script in Different Browsers
One of the key advantages of using Selenium is that it supports multiple browsers. To run the test script in different browsers, testers need to download the respective browser drivers and configure the environment variables accordingly. Once the drivers are installed, testers can use the same test script to run the tests on different browsers.
Getting started with Selenium requires installing and configuring the necessary software components, creating a basic test script, and running it in different browsers. With practice and experience, testers can become proficient in Selenium automation and use it to improve the quality and efficiency of their testing efforts.
Locators and Web Elements
A. Locators in Selenium
Locators are essential components of Selenium automation, as they help testers identify and interact with web elements on a web page. Locators are essentially a set of attributes that uniquely identify a web element on the page.
B. Different Types of Locators – ID, Name, Class, etc.
There are several types of locators that Selenium supports. Some of the commonly used locators include:
- ID: This is the most reliable and frequently used locator type in Selenium. It is a unique identifier for a web element on a page.
- Name: This locator type is used to identify elements based on the “name” attribute of the element.
- Class: This locator type is used to identify elements based on the “class” attribute of the element.
- XPath: This is a powerful locator type that allows testers to traverse the HTML structure of the page and identify elements based on their position in the DOM.
- CSS Selector: This locator type allows testers to identify elements based on their CSS attributes.
C. Locating Web Elements Using Different Locators
To locate a web element using a specific locator, testers can use the “findElement()” method provided by the WebDriver interface. For example, to locate an element using its ID, testers can use the following code:
WebElement element = driver.findElement(By.id("elementId"));
Similarly, to locate an element using its name or class, testers can use the following code:
WebElement element = driver.findElement(By.name("elementName"));
WebElement element = driver.findElement(By.className(“elementClass”));
To locate an element using XPath or CSS Selector, testers can use the following code:
WebElement element = driver.findElement(By.xpath("xpathExpression"));
WebElement element = driver.findElement(By.cssSelector(“cssSelectorExpression”));
D. Interacting with Web Elements
Once a web element is located, testers can interact with it using various methods provided by the WebElement interface. Some of the commonly used methods include:
- click(): This method is used to simulate a mouse click on the element.
- sendKeys(): This method is used to enter text into input fields or text areas.
- getText(): This method is used to retrieve the text content of the element.
- isEnabled(): This method is used to check if the element is enabled.
- isSelected(): This method is used to check if the element is selected.
By using the appropriate locators and interacting with web elements, testers can create powerful and reliable test scripts in Selenium.
Online Technical Job Support from India | 10+ Yrs. Exp. Mentor
API Testing with Postman technical support by 9 yrs. expert
Best Selenium C# Training Online with Live Project Hyderabad
Best Selenium Training Online with Live Project in Hyderabad
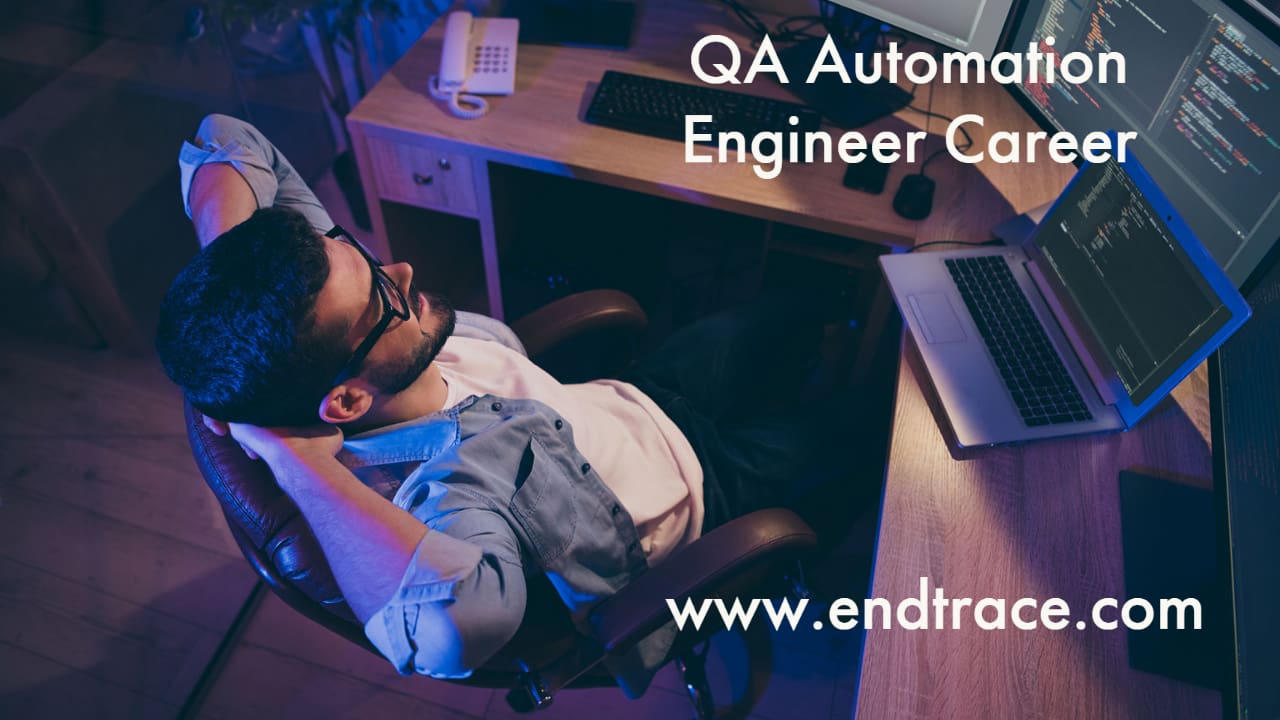
Handling Different Web Elements
A. Input Fields and Text Areas
1. Locating the Element – Use any of the locator types discussed earlier (ID, Name, Class, XPath, or CSS Selector) to locate the input field or text area element.
2. Interacting with the Element – Use the “sendKeys()” method to enter text into the input field or text area.
WebElement inputField = driver.findElement(By.id("inputFieldId"));
inputField.sendKeys(“Text to be entered”);
B. Buttons and Links
Locating the Element – Use any of the locator types discussed earlier (ID, Name, Class, XPath, or CSS Selector) to locate the button or link element.
Interacting with the Element – Use the “click()” method to simulate a mouse click on the button or link.
button.click();
C. Radio Buttons and Checkboxes
Locating the Element – Use any of the locator types discussed earlier (ID, Name, Class, XPath, or CSS Selector) to locate the radio button or checkbox element.
Interacting with the Element – Use the “click()” method to select or deselect the radio button or checkbox.
WebElement radioButton = driver.findElement(By.id(“radioButtonId”));
radioButton.click();
WebElement checkbox = driver.findElement(By.id(“checkboxId”));
checkbox.click();
D. Dropdowns and Lists
Locating the Element – Use any of the locator types discussed earlier (ID, Name, Class, XPath, or CSS Selector) to locate the dropdown or list element.
Interacting with the Element – Use the “Select” class provided by Selenium to interact with the dropdown or list element.
WebElement dropdown = driver.findElement(By.id("dropdownId"));
Select select = new Select(dropdown);
// Selecting by visible text
select.selectByVisibleText(“Option 1”);
// Selecting by index
select.selectByIndex(1);
// Selecting by value
select.selectByValue(“value1”);
E. Alerts and Popups
Alerts and Popups are commonly used in web applications to display important messages or notifications to users. They can interrupt the normal flow of the application and require user interaction to dismiss or take action on them. Selenium provides several methods to handle alerts and popups in test scripts.
1. Handling Alerts
Alerts are dialog boxes that appear on the screen with a message and require user action to proceed. They can be triggered by various events such as form submissions, input validation, or page redirects. To handle alerts in Selenium, testers can use the following steps:
- Switch to the alert dialog – The “switchTo()” method is used to switch the driver focus to the alert dialog.
Alert alert = driver.switchTo().alert();
- Retrieve the alert message – The “getText()” method is used to retrieve the message displayed in the alert dialog.
String alertMessage = alert.getText();
- Accept or dismiss the alert – The “accept()” or “dismiss()” method is used to accept or dismiss the alert dialog.
alert.accept(); // To accept the alert
alert.dismiss(); // To dismiss the alert
Here’s an example of how to handle an alert in Selenium:
// Locate the button that triggers the alert
WebElement button = driver.findElement(By.id("alertButtonId"));
// Click the button to trigger the alert
button.click();
// Switch to the alert dialog
Alert alert = driver.switchTo().alert();
// Retrieve the alert message
String alertMessage = alert.getText();
// Print the alert message
System.out.println(alertMessage);
// Accept the alert
alert.accept();
2. Handling Popups
Popups are new browser windows or tabs that open when a user performs a certain action, such as clicking on a link or a button. They can contain various types of content such as forms, images, or videos. To handle popups in Selenium, testers can use the following steps:
- Retrieve all open windows – The “getWindowHandles()” method is used to retrieve a set of all open windows in the browser.
Set<String> handles = driver.getWindowHandles();
- Switch to the desired window – The “switchTo()” method is used to switch the driver focus to the desired window by passing its window handle as a parameter.
String desiredWindow = "windowNameOrHandle";
driver.switchTo().window(desiredWindow);
Here’s an example of how to handle a popup in Selenium:
// Click on a link that opens a new window
WebElement link = driver.findElement(By.id(“popupLinkId”));
link.click();
// Retrieve all open windows
Set<String> handles = driver.getWindowHandles();
// Switch to the new window
for(String handle : handles) {
driver.switchTo().window(handle);
if(driver.getTitle().equals(“Popup Window Title”)) {
break;
}
}
// Perform actions on the popup window
// …
// Switch back to the main window
driver.switchTo().defaultContent();
By following these steps, testers can easily handle alerts and popups in their Selenium test scripts and ensure comprehensive test coverage.
Advanced Selenium Concepts
Selenium is an open-source testing framework that allows you to automate web browsers. In the previous sections, we discussed the basics of Selenium, how to get started with it, and how to interact with different web elements. In this section, we will discuss some advanced Selenium concepts, including working with frames and windows, handling implicit and explicit waits, different types of waits in Selenium, and synchronization in Selenium.
A. Working with Frames and Windows
When working with web pages, you may encounter frames and windows. A frame is an HTML document embedded inside another HTML document. A window is a top-level browsing context that represents an independent tab or window. Selenium provides APIs to interact with frames and windows.
To switch to a frame, you can use the switchTo()
method and pass the frame’s name, index, or WebElement. Here is an example:
driver.switchTo().frame("frameName");
To switch back to the default content, you can use the following method:
driver.switchTo().defaultContent();
To switch to a window, you can use the window()
method and pass the window’s name or handle. Here is an example:
driver.switchTo().window("windowName");
To close a window, you can use the close()
method. Here is an example:
driver.close();
B. Handling Implicit and Explicit Waits
In Selenium, waits are used to synchronize the test execution with the web page’s state. There are two types of waits: implicit waits and explicit waits.
Implicit waits are used to set a default waiting time for all web elements. The implicit wait time is set using the implicitlyWait()
method. Here is an example:
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
Explicit waits are used to wait for a specific web element to become visible, clickable, or have a specific value. The explicit wait time is set using the WebDriverWait
class. Here is an example:
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id(“elementId”)));
C. Different types of Waits in Selenium
There are different types of explicit waits in Selenium, including:
visibilityOfElementLocated(By by)
: waits for an element to be visible and present in the DOM.elementToBeClickable(By by)
: waits for an element to be clickable.textToBePresentInElementLocated(By by, String text)
: waits for a specific text to be present in an element.titleIs(String title)
: waits for a specific title of the web page.
D. Synchronization in Selenium
Synchronization is the process of ensuring that the test execution waits until the web page’s state is ready. In Selenium, synchronization can be achieved using waits.
By using waits, you can avoid synchronization errors, such as “ElementNotVisibleException,” “StaleElementReferenceException,” and “NoSuchElementException.” Waits ensure that the test waits for the web page’s state to become stable before performing an action.
advanced Selenium concepts, such as working with frames and windows, handling implicit and explicit waits, different types of waits in Selenium, and synchronization in Selenium, are crucial for creating robust and reliable test automation scripts. By using these concepts effectively, you can create efficient and stable test automation scripts that can help you save time and effort.
Selenium Automation Frameworks
A. What are Automation Frameworks?
Automation frameworks are pre-defined guidelines and standards that are used to create an organized and structured approach to automation testing. Automation frameworks provide a reusable set of guidelines and best practices that help in efficient and effective automation testing.
B. Types of Automation Frameworks – Keyword Driven, Data Driven, etc.
There are several types of automation frameworks used in the industry, including:
Keyword-driven framework: This framework uses a table of keywords and corresponding actions to automate test cases. It is best suited for applications that have a large number of test cases with varied input data.
Data-driven framework: This framework separates test data from the test scripts, which makes it easier to maintain and update test cases. It is best suited for applications that have a large amount of data to be tested.
Hybrid framework: This framework combines the best features of the keyword-driven and data-driven frameworks.
C. Selenium Frameworks – TestNG, JUnit, etc.
Selenium is an open-source automation testing tool that supports a wide range of programming languages such as Java, Python, Ruby, and C#. Selenium has its own set of automation frameworks that can be used to create automation test scripts. Some of the popular Selenium frameworks are:
TestNG: TestNG is a testing framework inspired by JUnit and NUnit, but with some additional functionalities. TestNG can be used to test all types of applications, including web, desktop, and mobile applications.
JUnit: JUnit is a popular testing framework that is widely used for unit testing in Java. It provides annotations for test methods, assertions, and test suites.
Cucumber: Cucumber is a behavior-driven development (BDD) framework that uses a natural language style to describe tests. Cucumber supports many programming languages, including Java, Ruby, and Python.
D. Creating a Selenium Test Automation Framework
Creating a Selenium test automation framework involves the following steps:
- Define the scope and objectives of the framework.
- Choose the automation framework type based on the application and requirements.
- Select the programming language and the Selenium framework that best suits the application.
- Design the framework architecture, including the test environment, test data, and test scripts.
- Develop the framework components such as test scripts, configuration files, and test data files.
- Integrate the test automation framework with the continuous integration and deployment (CI/CD) pipeline.
Test and validate the automation framework. - Maintain and update the automation framework as per the changes in the application or requirements.
Real-time Project with Selenium
Finally, we will discuss how to work on a real-time project with Selenium. We will learn how to analyze the project requirements, create test scenarios and cases, implement test automation, and analyze the test results using Selenium.
A. Understanding the project requirements
The first step towards working on a real-time project with Selenium is to understand the project requirements. This involves understanding the application, its functionality, the different user scenarios, and the expected outcomes. Once you have a clear understanding of the project requirements, you can proceed to the next step.
B. Creating Test Scenarios and Test Cases
Once you have a clear understanding of the project requirements, you need to create test scenarios and test cases. Test scenarios are high-level descriptions of the different user scenarios that need to be tested. Test cases, on the other hand, are detailed descriptions of the steps to be performed to test each scenario. You can create test scenarios and test cases using tools such as TestRail or Excel.
C. Implementing Test Automation with Selenium
Once you have created test scenarios and test cases, you can start implementing test automation with Selenium. This involves writing Selenium scripts to automate the test cases. You can use any of the Selenium frameworks such as TestNG, JUnit, or Cucumber to write the scripts.
You need to identify the different elements of the application using locators such as ID, name, class, or XPath, and perform the required actions on these elements using Selenium commands.
D. Running Test Cases and Analyzing Results
Once you have implemented test automation, you can start running the test cases and analyzing the results. You can run the test cases using tools such as Jenkins or CircleCI.
You can analyze the test results using tools such as TestRail, which provides detailed reports on the test results. You need to analyze the test results and identify the bugs or issues that need to be fixed.
Learn Selenium from Industry Expert:
If you’re looking to gain expertise in Selenium with Java, you can enroll in a comprehensive Selenium training course in Hyderabad. Our training is designed and delivered by industry experts with years of experience in Selenium automation testing.
During the course, you will learn how to use Selenium to create and execute test cases, handle different types of web elements, work with advanced concepts such as synchronization and waits, and implement automation frameworks using TestNG, JUnit, and other Selenium frameworks.
In addition to the training, we also offer career support to help you find the right opportunities and excel in your career as a Selenium automation tester. Our career support team provides guidance and assistance in preparing for interviews, building your resume, and connecting with potential employers.
To further enhance your skills and credentials, you can also opt for Selenium certifications, which validate your knowledge and expertise in Selenium automation testing. Our training course includes preparation for Selenium certification exams, so you can easily pass the exams and add a valuable certification to your resume.
Enroll in our Selenium with Java course today and take your career to new heights with comprehensive training and job support from industry experts.
Future of Selenium in Automation Testing
Selenium is an essential tool for web automation testing and has become the standard for web testing in the industry. As web applications continue to grow in complexity, the need for efficient and reliable testing tools like Selenium will only increase. With the rapid advancement of technology, it is expected that Selenium will continue to evolve and improve in the coming years.
Related Article to Read:
How to Become a QA Engineer: A Step-by-Step Guide
Brief about Selenium — Getting started with Selenium Automation Testing
Exploring Maven Repository in Selenium Java – QA Automation
Manual Testing Job Support Online | 12 yrs QA Testing Expert
Selenium with Java Technical Help online from Industry Expert
Automation Testing — Selenium career scope and salary | Training
Related Articles
Are You Looking for Digital Marketing Projects for Best Practice?
You've aced your digital marketing course. Now what? The real challenge begins: gaining practical, hands-on experience. Theory alone won't cut it in...
How to Start a Digital Marketing Projects for Best Practice – Ultimate Guide
Starting a digital marketing project can seem overwhelming, but with a clear, step-by-step guide, you can navigate this journey with ease. Embarking...
Drive Traffic with Organic CTR: SEO Ranking Tips for Website
Introduction: Are you struggling to get your website to rank higher in search results? Are you frustrated by the lack of traffic your website is...
Crack the SEO Interview Q&A | Boost Your Skills & Poise
In our previous article, we covered 20 Technical SEO Interview questions and answers for aspiring SEO professionals. In this article, we will be...
How to do step-by-step SEO Competitor Analysis + Template
Introduction A. Importance of SEO competitor analysis In today's digital landscape, search engine optimization (SEO) has become a critical component...
Ace Your Technical SEO Interview: Strategies for Learner
1. Introduce about yourself in brief Answer: My name is [Your Name], I am a recent graduate with a Bachelor's degree in Marketing. Throughout my...