Introduction:
Selenium WebDriver is an open-source tool for automating web browsers. It provides a simple and intuitive interface for controlling web browsers and performing tasks such as navigating to websites, interacting with elements on a page, and verifying expected outcomes.
In today’s fast-paced world, automating web browser tasks has become increasingly important for businesses, developers, and testers. The ability to handle multiple tabs in a browser is a key component of effective automation testing, as it enables you to perform complex interactions and comparisons across multiple pages. By handling multiple tabs, you can automate tasks that would be time-consuming and error-prone to do manually.
In this article, we will explore how to handle multiple tabs in Selenium WebDriver and the benefits of taking a Selenium Java course. We will also discuss the importance of hands-on practice and finding a training program taught by an industry expert.
Understanding window handles:
In Selenium, a window handle is a unique identifier for a browser window or tab. When a new tab is opened in a browser, Selenium automatically assigns it a unique window handle. This handle can be used to switch between tabs, making it possible to interact with multiple tabs in a single test.
When you first launch a browser session with Selenium, it automatically captures the window handle of the default tab. To access additional tabs, you must first retrieve a list of all open window handles and then switch to the desired tab using its handle.
Here’s an example of how to retrieve a list of window handles in Selenium WebDriver using Java:
List<String> handles = driver.getWindowHandles();
Once you have a list of window handles, you can use the driver.switchTo().window() method to switch between tabs. For example:
for (String handle : handles) {
driver.switchTo().window(handle);
// perform actions on the current tab
}
By using window handles in Selenium WebDriver, you can easily switch between tabs, interact with elements on each tab, and verify expected outcomes. This makes it possible to automate complex tasks that would be time-consuming and error-prone to do manually.
Steps for handling multiple tabs in Selenium WebDriver:
Handling multiple tabs in Selenium WebDriver requires a few simple steps. Here’s an overview of the process:
Step 1: Get a list of all open tabs To get a list of all open tabs in a browser session, you can use the driver.getWindowHandles() method. This method returns a list of all window handles associated with the current browser session.
Step 2: Iterate over the list of window handles Once you have a list of window handles, you can use a for loop to iterate over the list. For each handle in the list, you can use the driver.switchTo().window(handle) method to switch to the corresponding tab.
Step 3: Switch to each tab and interact with it as needed Once you have switched to a specific tab using its handle, you can interact with elements on the page, perform actions, and verify expected outcomes. After completing your actions on one tab, you can switch to the next tab using the same process.
Here’s an example of how to handle multiple tabs in Selenium WebDriver using Java:
List<String> handles = driver.getWindowHandles();
for (String handle : handles) {
driver.switchTo().window(handle);
// perform actions on the current tab
}
By following these steps, you can easily handle multiple tabs in Selenium WebDriver and automate complex tasks that would be time-consuming and error-prone to do manually.
Example code in Java
Here’s an example of how to handle multiple tabs in Selenium WebDriver using Java:
import java.util.List;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.chrome.ChromeDriver;
public class HandleMultipleTabs {
public static void main(String[] args) {
// set up web driver
System.setProperty(“webdriver.chrome.driver”, “/path/to/chromedriver”);
WebDriver driver = new ChromeDriver();
// open multiple tabs
driver.get(“https://www.google.com”);
driver.findElement(By.cssSelector(“body”)).sendKeys(Keys.CONTROL + “t”);
driver.get(“https://www.yahoo.com”);
driver.findElement(By.cssSelector(“body”)).sendKeys(Keys.CONTROL + “t”);
// get a list of window handles
List<String> handles = driver.getWindowHandles();
// iterate over the list of handles and switch to each tab
for (String handle : handles) {
driver.switchTo().window(handle);
System.out.println(“Current URL: ” + driver.getCurrentUrl());
}
// close the browser
driver.quit();
}
}
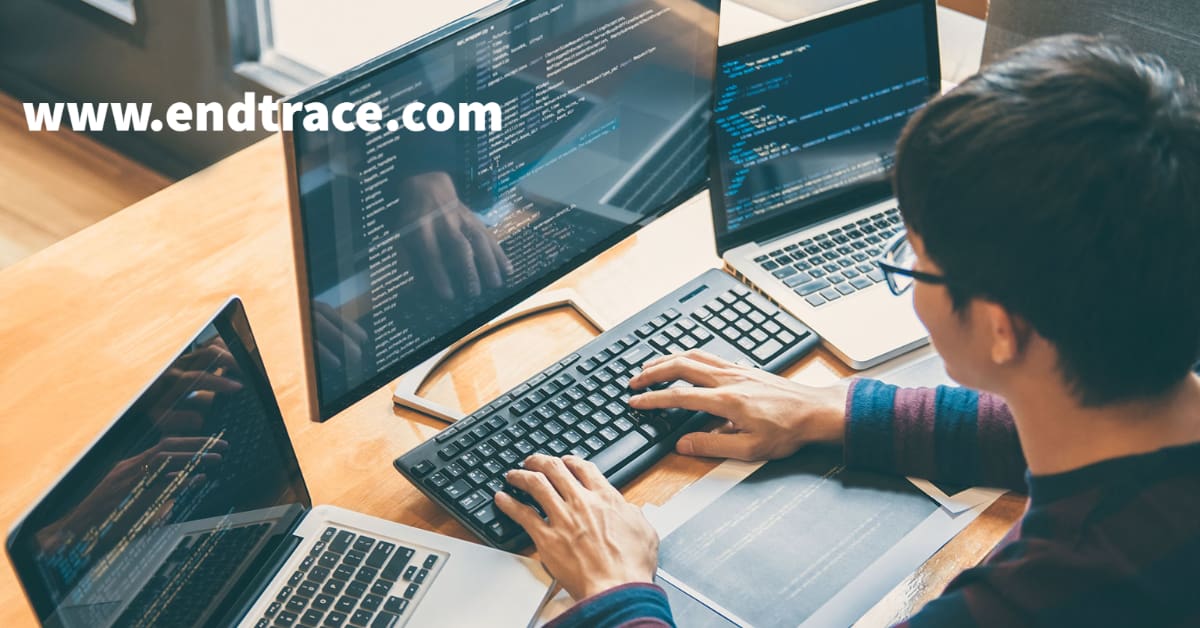
In this example, the HandleMultipleTabs class sets up a ChromeDriver instance and opens multiple tabs to Google and Yahoo. The program then retrieves a list of window handles using driver.getWindowHandles() and iterates over the list, switching to each tab using the driver.switchTo().window(handle) method. Finally, the program prints the URL of the current tab to demonstrate that it has switched between tabs.
This is just one example of how to handle multiple tabs in Selenium WebDriver using Java. By using window handles and the switchTo() method, you can automate complex tasks that involve switching between multiple tabs in a browser.
Common challenges while handling multiple tabs:
Handling multiple tabs in Selenium WebDriver can present some challenges, but with the right approach and tools, these challenges can be overcome. Some common challenges include:
• Managing multiple window handles: Keeping track of multiple window handles and switching between them can be a challenge, especially if the number of tabs is large.
• Interacting with dynamic content: When working with dynamic content, it can be difficult to accurately interact with the right tab.
• Synchronizing between tabs: Ensuring that the automation tests run smoothly and synchronize correctly between tabs can be a challenge.
• To overcome these challenges, it is important to have a clear understanding of window handles and to use best practices for handling multiple tabs in Selenium WebDriver.
Additionally, implementing tools such as wait statements can help ensure that the automation tests run smoothly and synchronize correctly between tabs.
API Testing with Postman technical support by 9 yrs. expert
Manual Testing Job Support Online | 12 yrs QA Testing Expert
Automation testing Job Support
Online Automation Testing Job Support
Best practices for handling multiple tabs:
Handling multiple tabs effectively in Selenium WebDriver requires a systematic approach and adherence to certain best practices. Some of these best practices include:
• Store all window handles in a list: To effectively switch between tabs, it is important to store all window handles in a list and keep track of the current handle.
• Use a wait statement: Using a wait statement before interacting with a new tab can help ensure that the content on the new tab is fully loaded before attempting to interact with it.
• Interact with each tab: To effectively test each tab, it is important to switch to each tab and interact with it as needed.
• Synchronize between tabs: To ensure that the automation tests run smoothly and synchronize correctly between tabs, it is important to use techniques such as explicit waits and webdriver waits.
By following these best practices, you can effectively handle multiple tabs in Selenium WebDriver and ensure that your automation tests run smoothly and accurately.
How to Become a QA Engineer: A Step-by-Step Guide
Exploring Maven Repository in Selenium Java – QA Automation
How to switch from manual tester to automation Testing and Benefits
Learn Automation Testing – Become a great Selenium Testing Engineer
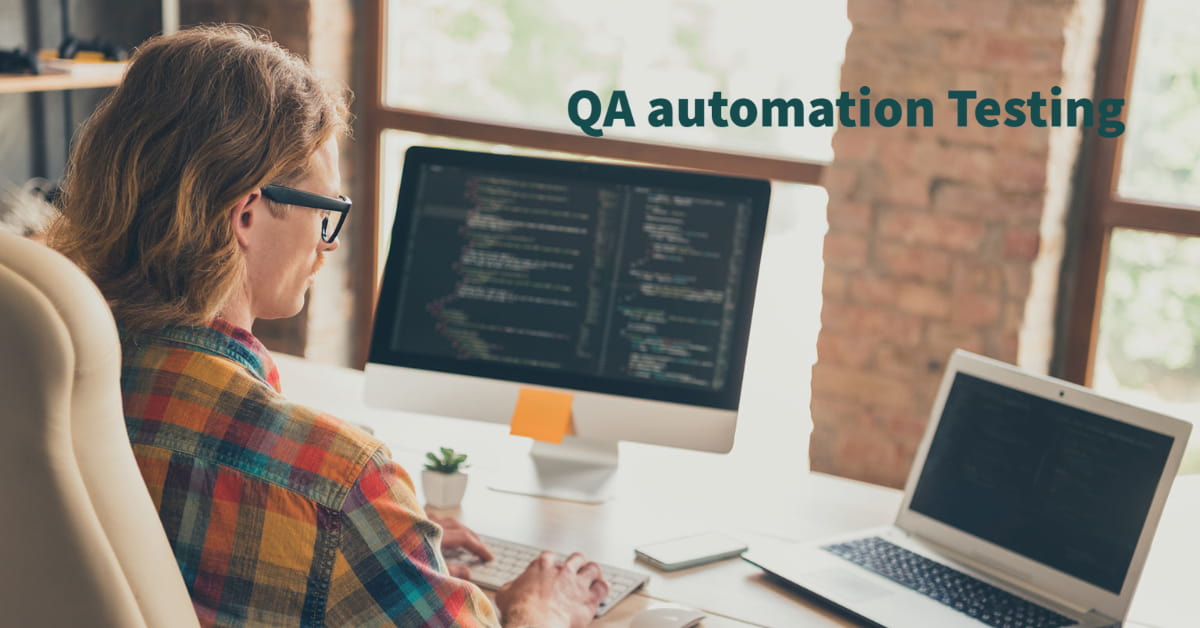
Troubleshooting tips for handling multiple tabs in Selenium WebDriver:
- Incorrect window handle: If you receive an error message that the window handle is incorrect, it’s likely that the handle has changed. Try refreshing the list of window handles and switching to the correct one.
- Stale element reference: If you receive a “stale element reference” error message, it means that the element you are trying to interact with is no longer attached to the DOM. You can resolve this issue by using explicit wait statements and synchronizing your test with the page.
- Inconsistent window titles: If you are using window titles to switch between tabs, be aware that they may change dynamically. To avoid this issue, you can use window handles instead.
- Slow page load times: Slow page load times can cause issues when switching between tabs. To avoid this, use implicit wait statements to wait for the page to fully load before switching tabs.
The Selenium with Java training provided by industry experts will provide you with the technical help you need to succeed in your career. Additionally, a Selenium Java training course with practice will allow you to apply the concepts you have learned and develop your problem-solving skills. Story: How a Manual Tester become QA Automation Expert in 3 Months
Advantages of Selenium Java Training at Endtrace
Selenium Java training with practice is an effective way to develop the skills needed to become an expert in automation testing. Here are some of the advantages of taking a training from Endtrace that includes both theory and practice:
Hands-on Experience: Practical experience is essential for learning Selenium WebDriver. By taking a Selenium Java training program with practice, you will have the opportunity to work on real-world projects and apply the concepts and techniques you have learned.
Deep Understanding: Hands-on experience helps you to develop a deeper understanding of Selenium WebDriver and its applications. With practice, you will be able to identify and solve complex problems more efficiently. When learning to become a smart automation testing engineer, if we don’t talk about the test automation tools, then we are doing an injustice to the industry.
Confidence Building: Practical experience also helps to build confidence in your skills and abilities. By working on real projects and seeing the results of your efforts, you will become more confident in your ability to use Selenium WebDriver for automation testing.
Career Advancement: A training program that includes both theory and practice can help you to advance in your career. With practical experience, you will be able to demonstrate your skills to potential employers and stand out in a competitive job market. How to Become a QA Engineer: A Step-by-Step Guide
mastering the skills of handling multiple tabs in Selenium WebDriver and taking a Selenium Java training program can set you apart as a skilled and knowledgeable automation tester. So, if you are looking to advance in this field, make sure to invest in your education and skill development.
Conclusion:
Handling multiple tabs in Selenium WebDriver is an essential skill for automation testing. With the knowledge of window handles and the switchTo() method, you can easily switch between tabs and automate complex tasks. Whether you’re a beginner or an experienced Selenium Java user, honing your skills in handling multiple tabs is important for your success as an automation tester.
And if you want to learn more about Selenium Java, consider enrolling in a Selenium Java Course or taking a Selenium Java Training with Practice. With the help of a Selenium Java Training by Industry Expert, you can master the ins and outs of Selenium WebDriver and become a highly skilled automation tester.
Related Article to Read:
What is Web Application Testing? Important points to consider while Testing
API Testing with Postman technical support by 9 yrs. expert
Online Technical Job Support from India | 10+ Yrs. Exp. Mentor
Best practices for maintaining testing framework using Java Selenium webdriver
Manual Testing Job Support Online | 12 yrs QA Testing Expert
Learn Automation Testing — Become a great Selenium Testing Engineer
Automation testing Job Support
Online Automation Testing Job Support
Related Articles
Are You Looking for Digital Marketing Projects for Best Practice?
You've aced your digital marketing course. Now what? The real challenge begins: gaining practical, hands-on experience. Theory alone won't cut it in...
How to Start a Digital Marketing Projects for Best Practice – Ultimate Guide
Starting a digital marketing project can seem overwhelming, but with a clear, step-by-step guide, you can navigate this journey with ease. Embarking...
Drive Traffic with Organic CTR: SEO Ranking Tips for Website
Introduction: Are you struggling to get your website to rank higher in search results? Are you frustrated by the lack of traffic your website is...
Crack the SEO Interview Q&A | Boost Your Skills & Poise
In our previous article, we covered 20 Technical SEO Interview questions and answers for aspiring SEO professionals. In this article, we will be...
How to do step-by-step SEO Competitor Analysis + Template
Introduction A. Importance of SEO competitor analysis In today's digital landscape, search engine optimization (SEO) has become a critical component...
Ace Your Technical SEO Interview: Strategies for Learner
1. Introduce about yourself in brief Answer: My name is [Your Name], I am a recent graduate with a Bachelor's degree in Marketing. Throughout my...